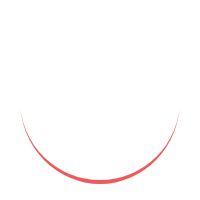
In the world of object-oriented programming, encapsulation plays a vital role in achieving code organization, data protection, and overall software design. One key element of encapsulation is the encapsulation operator, which allows programmers to control the access to internal components of a class or object. In this article, we will explore the encapsulation operator and its significance in software development.
What is encapsulation?
Encapsulation refers to the bundling of data and methods within a class, where the data is hidden from direct access by external entities. It is a fundamental principle in object-oriented programming (OOP) that promotes the concept of data abstraction and information hiding.
Importance of encapsulation in programming
Encapsulation plays a crucial role in building robust and maintainable software systems. By encapsulating data and behavior within classes, developers can create modular, reusable, and secure code.
Definition and Explanation
Encapsulation in object-oriented programming
In the realm of object-oriented programming, encapsulation is the process of binding data and methods together within a class, forming an encapsulated unit. This unit acts as a black box, exposing only a limited set of well-defined interfaces to interact with the internal components.
Encapsulation operator
The encapsulation operator is a mechanism provided by programming languages to control the visibility and accessibility of internal components within a class. It allows programmers to specify which members of a class are accessible from outside the class and which are hidden, thus enforcing encapsulation.
Role of encapsulation in data hiding
One of the primary purposes of encapsulation is to achieve data hiding. By encapsulating data within a class, it becomes inaccessible to external entities, preventing unauthorized modifications. This enhances data security and integrity, crucial aspects in software development.
Encapsulation and information hiding
Although encapsulation and information hiding are closely related, they are not synonymous. Information hiding is a broader concept that encompasses encapsulation. Encapsulation is concerned with bundling related data and behavior together, while information hiding focuses on selectively exposing or hiding internal details to ensure proper abstraction.
Encapsulation offers several benefits that contribute to the development of efficient and maintainable software systems.
Modularity and code organization
Encapsulation promotes modularity by encapsulating related data and behavior within classes. This allows developers to break down complex systems into smaller, more manageable components. Each class encapsulates a specific set of functionalities, making the codebase easier to understand, maintain, and modify.
Data protection and security
Encapsulation ensures data protection by hiding the internal state of an object and providing controlled access through well-defined interfaces. By encapsulating data, developers can prevent unauthorized modifications or access to sensitive information. This enhances the security and integrity of the software.
Code reusability
Encapsulation promotes code reusability by encapsulating data and behavior within classes. Once a class is defined, it can be reused in different parts of the program or in other projects without modification. This saves time and effort in writing duplicate code and improves overall code efficiency.
Encapsulation enhances flexibility and maintainability by isolating changes within the encapsulated class. When modifications or updates are required, developers can focus on the specific class without affecting other parts of the system. This reduces the risk of introducing bugs or unintended consequences, making maintenance and updates more efficient.
Implementing Encapsulation
Programming languages use access specifiers, such as public, private, and protected, to control the visibility and accessibility of class members. By specifying access levels, developers can enforce encapsulation rules and restrict direct access to internal components. Public members are accessible from anywhere, while private members are only accessible within the class itself. Protected members are accessible within the class and its subclasses.
Examples of encapsulation in programming languages
Different programming languages provide varying mechanisms for implementing encapsulation. For example, in Java, you can use the private access specifier to hide variables and methods from external access. In C++, you can use access specifiers like private, public, and protected to define the visibility of class members. Each language has its own syntax and conventions for achieving encapsulation.
Encapsulation in real-world scenarios
Encapsulation is not limited to the realm of programming languages; it also has real-world applications. For example, in electrical engineering, encapsulation is used to protect sensitive components within a circuit by enclosing them in a protective casing. This prevents external interference and damage, ensuring the proper functioning of the circuit.
Encapsulation in software design patterns
Software design patterns, such as the Model-View-Controller (MVC) pattern, heavily rely on encapsulation. In the MVC pattern, the model encapsulates the application's data and business logic, the view encapsulates the user interface components, and the controller encapsulates the interaction between the model and the view. This separation of concerns enhances code organization, maintainability, and scalability.
Best Practices for Encapsulation
To make the most out of encapsulation, it is important to follow some best practices:
Encapsulating data and behavior
Ensure that data and behavior relevant to a specific class are encapsulated within that class. This minimizes dependencies and improves code clarity.
Limiting direct access to internal components
Use appropriate access specifiers to limit direct access to internal components. This prevents external entities from modifying the internal state in unexpected ways, enhancing data security and integrity.
Defining clear and consistent interfaces
Design classes with well-defined interfaces that encapsulate the essential functionality. This promotes code clarity, reusability, and ease of maintenance.
What is the difference between encapsulation and abstraction?
Encapsulation and abstraction are related but distinct concepts. Encapsulation refers to bundling data and behavior within a class, while abstraction focuses on presenting a simplified and generalized view of an object. Encapsulation enforces access controls, while abstraction hides unnecessary implementation details.
Can encapsulation be achieved in procedural programming?
Encapsulation is more commonly associated with object-oriented programming, where it is a core principle. However, procedural programming languages can also achieve a limited form of encapsulation through the use of modules or namespaces to group related functions and variables.
How does encapsulation contribute to code maintainability?
Encapsulation improves code maintainability by localizing changes within encapsulated classes. Modifying the internal implementation of a class does not require modifying other parts of the codebase, reducing the risk of introducing bugs and making maintenance more efficient.
Is encapsulation the same as information hiding?
Encapsulation and information hiding are closely related but not identical. Encapsulation focuses on bundling data and behavior within a class, while information hiding is the practice of selectively exposing or concealing internal details. Encapsulation is one mechanism for achieving information hiding.
Can encapsulation be overridden in inheritance?
Inheritance does not override encapsulation. When a class is inherited, the encapsulated members retain their access specifiers and visibility rules. Subclasses can access protected and public members of their base class but cannot directly access private members. Encapsulation remains intact even in inheritance scenarios.