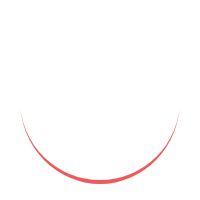
Inspection operators are a critical component of programming languages and play a vital role in code optimization, troubleshooting, and debugging. In this article, we'll explore the inspection operator, what it is, and how it works.
What is an Inspection Operator?
An inspection operator is a programming construct used to query and analyze the value of an expression. Inspection operators are used to determine the type, size, and value of an expression, making them valuable in a wide range of programming tasks. Inspection operators are available in most modern programming languages, including Java, Python, Ruby, and C++.
How Does an Inspection Operator Work?
Inspection operators work by evaluating an expression and returning information about that expression. For example, the typeof operator in JavaScript can be used to determine the data type of a given variable. The operator evaluates the variable and returns a string indicating its data type, such as "string", "number", or "boolean".
Inspection Operator Types of Inspection Operators
There are three main types of inspection operators: unary, binary, and ternary.
Unary Inspection Operators
Unary inspection operators take a single operand and return a value based on that operand. Examples of unary inspection operators include typeof in JavaScript, sizeof in C++, and is_integer in Python.
inary Inspection Operators
Binary inspection operators take two operands and return a value based on the relationship between those operands. Examples of binary inspection operators include == (equality) and != (inequality) in most programming languages, as well as is and in operators in Python.
Ternary Inspection Operators
Ternary inspection operators take three operands and return a value based on the relationship between those operands. The most common ternary operator is the conditional operator (?:), which is used to assign a value to a variable based on a condition.
Common Use Cases for Inspection Operators
Inspection operators are used in a wide range of programming tasks, including:
Checking the type of a variable
Determining the size of an array or string
Checking if a variable is null or undefined
Checking if a variable is a specific value or meets a certain condition
Converting data types
Debugging programs
Benefits of Using Inspection Operators
The use of inspection operators can offer several benefits, including:
Improved code readability and organization
Enhanced debugging capabilities
More efficient programming
Better error handling and prevention
Disadvantages of Using Inspection Operators
While inspection operators offer many benefits, they also have some disadvantages, including:
Increased complexity
Reduced performance in some cases
Potential for errors if not used correctly
Real-World Examples of Inspection Operators
Inspection operators are used in a wide range of programming tasks. Here are some examples of inspection operators in action:
Checking if a variable is null or undefined before accessing it in JavaScript
Checking the length of a string or array in Python using the len() function
Checking if two objects are equal using the == operator in Java
Converting a string to an integer using the int() function in Python
Best Practices for Using
Best Practices for Using Inspection Operators
To get the most out of inspection operators, it's essential to follow some best practices. Here are some tips for using inspection operators effectively:
Understand the syntax and semantics of the operator you're using. Read the documentation carefully to ensure that you're using the operator correctly and that you understand the results it returns.
Use inspection operators judiciously. Don't rely on inspection operators too heavily or use them unnecessarily. In some cases, there may be more efficient or readable ways to achieve the same result.
Keep your code organized and readable. Use indentation and appropriate formatting to make your code easy to read and understand. Use comments to explain the purpose and logic of your code, especially if you're using inspection operators in complex or unusual ways.
Test your code thoroughly. Use automated testing tools to ensure that your code is working as expected. Test your code under a variety of conditions, including edge cases and unexpected inputs.
Stay up to date with new developments and best practices in programming. Follow industry blogs and attend conferences and workshops to learn about new tools and techniques for using inspection operators and other programming constructs.
How to Debug Programs with Inspection Operators
Inspection operators are an essential tool for debugging programs. By using inspection operators to check the value and type of variables at various points in your code, you can identify problems and errors more quickly and efficiently. Here are some tips for using inspection operators for debugging:
Use inspection operators to check the value and type of variables at key points in your code. By checking the value and type of variables as they change, you can identify where and when errors occur.
Use conditional operators to set breakpoints and control program flow. By using conditional operators, you can create conditional breakpoints that pause the execution of your program when certain conditions are met. This can help you identify the source of errors and bugs more quickly.
Use inspection operators to log messages to the console or a log file. By logging messages to the console or a log file, you can get a better understanding of how your program is running and identify potential errors or issues.
Common Pitfalls When Using Inspection Operators
Using inspection operators can be tricky, and there are some common pitfalls to watch out for. Here are some common mistakes to avoid when using inspection operators:
Using the wrong operator. Make sure that you're using the correct operator for the task at hand. Using the wrong operator can result in incorrect or unexpected results.
Not checking for null or undefined values. Before accessing a variable, make sure that it's not null or undefined. Failure to do so can result in errors and crashes.
Using inspection operators too often. Overusing inspection operators can slow down your program and make it harder to read and understand.
How to Choose the Right Inspection Operator
Choosing the right inspection operator depends on the task at hand. Here are some tips for choosing the right inspection operator:
Determine what information you need to extract from the expression. Do you need to know the type, size, or value of the expression? Different inspection operators provide different information.
Consider the syntax and semantics of the operator. Make sure that you understand how the operator works and what results it returns.
Consider the performance implications of the operator. Some inspection operators are more efficient than others, so consider the performance implications of using a particular operator.
Future of Inspection Operators
As programming languages continue to evolve, inspection operators are likely to become even more powerful and versatile. New inspection operators may be developed to address specific programming challenges, and existing operators may be enhanced to provide more information and better performance.
Inspection Operator FAQs
What are inspection operators?
Inspection operators are programming constructs that allow programmers to extract information about variables and expressions at runtime.
What are some common inspection operators?
Common inspection operators include typeof, instanceof, and debugger.
How can I use inspection operators for debugging?
By using inspection operators to check the value and type of variables at key points in your code, you can identify problems and errors more quickly and efficiently.
What are some best practices for using inspection operators?
Best practices for using inspection operators include understanding the syntax and semantics of the operator, using inspection operators judiciously, keeping your code organized and readable, testing your code thoroughly, and staying up to date with new developments in programming.
How can I choose the right inspection operator for my task?
To choose the right inspection operator, consider what information you need to extract from the expression, consider the syntax and semantics of the operator, and consider the performance implications of the operator.