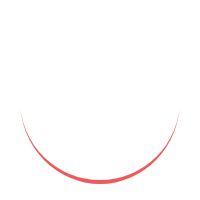
Have you ever wondered how computer programs organize and arrange data in a specific order? Sorting operators play a crucial role in the realm of computer science and programming, enabling efficient data manipulation. In this article, we will explore the concept of sorting operators, their importance, common types, implementation, benefits, best practices, and more. So, let's dive in and discover the world of sorting operators!
Sorting Operator Introduction
In the digital age, data is abundant, and the ability to sort and organize it efficiently is of utmost importance. Sorting operators serve as powerful tools in achieving this goal. Whether you are working with a small dataset or handling massive amounts of information, sorting operators provide a systematic approach to arranging data elements.
What is a Sorting Operator?
A sorting operator is a programming construct or function that arranges data elements in a specific order. It takes an input sequence and produces an output sequence in which the elements are sorted based on a defined criterion. Sorting operators are commonly used in various programming languages and databases to facilitate tasks like searching, filtering, and organizing data for efficient retrieval.
Importance of Sorting Operators
Sorting operators offer several benefits and are integral to many applications. Here are some key reasons why sorting operators are important:
Efficient Searching: Sorting data allows for efficient searching algorithms like binary search, which can significantly speed up the retrieval process. By organizing data in a specific order, it becomes easier to locate desired elements quickly.
Improved Performance: Sorting operators optimize the performance of algorithms that rely on sorted data, such as merge sort or quicksort. These algorithms exhibit better time complexity when the input data is already sorted or partially sorted.
Data Analysis: Sorting operators play a crucial role in data analysis tasks, such as finding the largest or smallest values, identifying patterns, or grouping data based on specific attributes. They enable efficient exploration and extraction of valuable insights from datasets.
Common Sorting Operators
There are several common types of sorting operators used in programming languages. These include:
1. Bubble Sort
Bubble sort is a simple and intuitive sorting algorithm that repeatedly compares adjacent elements and swaps them if they are in the wrong order. Although not the most efficient algorithm for large datasets, it is easy to understand and implement.
2. Insertion Sort
Insertion sort works by iteratively inserting elements from an unsorted part of the array into their correct positions within a sorted portion. It is efficient for small datasets or nearly sorted arrays.
3. Selection Sort
Selection sort divides the input array into two parts: the sorted part and the unsorted part. It repeatedly selects the minimum element from the unsorted part and places it at the beginning of the sorted part.
4.Sorting Operator
Merge sort is a divide-and-conquer algorithm that recursively divides the input array into smaller subarrays, sorts them, and then merges them back together to obtain the final sorted array. It is known for its stability and consistent performance.
5. Quick Sort
Quick sort is another divide-and-conquer algorithm that partitions the input array based on a pivot
point, and recursively sorts the subarrays on either side of the pivot. It is widely used and known for its efficiency in practice.
How Sorting Operators Work
Sorting operators follow specific algorithms or logic to arrange data elements in a desired order. The exact implementation may vary depending on the sorting operator and the programming language being used. However, the general steps involved in sorting typically include:
Comparing Elements: The sorting operator compares pairs of elements to determine their relative order based on the specified criterion.
Swapping Elements: If the compared elements are in the wrong order, the sorting operator swaps them to correct their positions.
Iterating and Repeating: The process of comparing and swapping is repeated until the entire sequence is sorted.
Returning Sorted Sequence: Once the sorting process is complete, the operator returns the sorted sequence.
Different sorting algorithms may have variations in these steps, but the underlying principles remain consistent.
Benefits of Using Sorting Operators
Using sorting operators in your programs can bring several benefits:
Efficiency: Sorting operators provide efficient ways to organize and retrieve data, leading to improved performance and faster processing times.
Simplicity: Many sorting operators have straightforward implementations, making them easy to understand and use even for beginners in programming.
Flexibility: Sorting operators can be customized to sort data based on different criteria, such as alphabetical order, numerical value, or user-defined rules.
Reusability: Once implemented, sorting operators can be reused in multiple applications or scenarios, saving time and effort in coding.
Examples of Sorting Operators in Different Programming Languages
Let's explore some examples of sorting operators in different programming languages:
Python:
python
Copy code
# Using the built-in sorting operator
numbers = [5, 2, 8, 1, 9]
numbers.sort()
print(numbers)
JavaScript:
javascript
Copy code
// Using the sort() method
let fruits = ['apple', 'banana', 'cherry', 'date', 'elderberry'];
fruits.sort();
console.log(fruits);
Java:
java
Copy code
// Using the Arrays.sort() method
int[] array = {4, 2, 9, 1, 7};
Arrays.sort(array);
System.out.println(Arrays.toString(array));
These examples demonstrate how sorting operators can be implemented in different languages to sort arrays or lists.
Best Practices for Using Sorting Operators
To make the most of sorting operators, consider the following best practices:
Choose the Right Algorithm: Understand the characteristics and performance of different sorting algorithms to select the most suitable one for your specific use case.
Consider Input Size: Be aware of the time complexity of the chosen sorting algorithm and consider its efficiency for handling the expected size of the input data.
Handle Edge Cases: Account for special cases, such as empty arrays or already sorted data, to optimize the sorting process and avoid unnecessary operations.
Optimize for Stability: If the order of equal elements is important, choose a stable sorting algorithm that preserves the relative order of equal elements during the sorting process.
Test and Benchmark: Test your sorting implementation with various input sizes and compare its performance against other algorithms to ensure it meets your requirements.
Sorting Operator Performance Considerations
While sorting operators can greatly enhance data organization, it's important to consider their performance implications:
Time Complexity: Different sorting algorithms have varying time complexities, which impact the efficiency of sorting. Consider the size of the dataset and choose an algorithm accordingly.
Space Complexity: Some sorting algorithms require additional memory space for temporary storage or auxiliary arrays. Be mindful of the memory requirements, especially when dealing with large datasets.