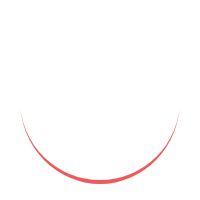
C++ is a versatile and powerful programming language that has been widely used for decades. Developed in the early 1980s, it has evolved to become a popular choice among developers for various applications. This article provides an in-depth exploration of C++, its features, programming basics, object-oriented programming, memory management, the Standard Template Library (STL), and its applications.
History and Development of C++
C++ was created by Bjarne Stroustrup as an extension of the C programming language. Stroustrup wanted to enhance C by adding support for object-oriented programming while retaining its efficiency and low-level access to memory. The first version of C++ was released in 1983, and since then, it has undergone several revisions, with the latest standardized version known as C++20.
Key Features of C++
C++ incorporates several key features that make it a powerful programming language:
Object-Oriented Programming:
C++ supports object-oriented programming (OOP) principles, allowing developers to organize code into reusable objects. This paradigm promotes modularity, code reusability, and easy maintenance.
Low-Level Access to Memory:
C++ provides low-level access to memory through pointers, allowing developers to manipulate memory directly. This feature is particularly useful when dealing with hardware interfaces, system programming, and optimizing performance-critical code.
Portability and Efficiency:
C++ is a highly portable language, allowing developers to write code that can be executed on different platforms. It also emphasizes efficiency, giving programmers control over system resources and enabling them to write optimized code.
Basics of C++ Programming
To get started with C++, understanding its basic concepts is essential. Here are some fundamental aspects:
Variables and Data Types:
In C++, variables are used to store and manipulate data. C++ supports various data types, including integers, floating-point numbers, characters, booleans, and more. Variables must be declared with a specific type before they can be used.
Control Structures:
Control structures such as if-else statements, loops, and switch statements allow developers to control the flow of program execution based on certain conditions. They help in making decisions and repeating code as necessary.
Functions:
Functions in C++ are blocks of code that perform specific tasks. They allow for code modularity and reusability by breaking down complex tasks into smaller, manageable parts.
Object-Oriented Programming in C++
One of the significant advantages of C++ is its support for object-oriented programming. This paradigm allows developers to create classes, objects, and hierarchies, resulting in clean and modular code. Some important concepts include:
Classes and Objects:
Classes are user-defined data types that encapsulate data and functions. Objects are instances of classes, representing specific entities or concepts. They allow for data abstraction and provide a blueprint for creating multiple objects.
Inheritance and Polymorphism:
Inheritance allows classes to inherit properties and behaviors from other classes, enabling code reuse and specialization. Polymorphism allows objects of different classes to be treated as instances of a common superclass, enhancing flexibility and extensibility.
Encapsulation and Data Hiding:
Encapsulation involves bundling data and functions together into a single unit (class), protecting data from external access. Data hiding further restricts direct access to data, promoting data integrity and security.
Memory Management in C++
Efficient memory management is crucial in C++ programming. Understanding the following concepts will help in utilizing memory effectively:
Pointers and References:
Pointers are variables that store memory addresses. They allow direct manipulation of memory, facilitating dynamic memory allocation and resource management. References, on the other hand, provide an alias or alternative name for an existing object.
Dynamic Memory Allocation:
C++ provides mechanisms for dynamically allocating and deallocating memory using operators such as new and delete. This feature is useful when creating data structures of varying sizes or when memory requirements change during program execution.
Standard Template Library (STL)
The Standard Template Library (STL) is a powerful component of C++ that provides ready-to-use data structures and algorithms. It simplifies complex tasks and enhances code readability and maintainability. The key components of the STL include:
Containers:
STL containers are data structures that store and organize objects efficiently. Examples include vectors, lists, queues, and maps, each designed for specific use cases.
Algorithms:
STL algorithms are a collection of functions that operate on containers. They enable common operations like searching, sorting, and modifying container elements. By utilizing these algorithms, developers can avoid reinventing the wheel and focus on solving higher-level problems.
Iterators:
STL iterators provide a way to access and traverse elements in containers. They act as a generalized pointer, allowing for efficient and flexible data manipulation.
C++ for Application Development
C++ finds application in various domains due to its versatility and performance. Some notable areas where C++ excels are:
Graphics and Game Development:
C++ is widely used in the gaming industry to create high-performance graphics engines and game logic. Its low-level control and efficient memory management make it an ideal choice for resource-intensive applications.
System Programming:
C++ is extensively used for system programming, enabling developers to interact with the operating system and hardware interfaces directly. It provides the necessary tools to build operating systems, drivers, and other system-level software.
Embedded Systems:
C++ is commonly used in embedded systems development, where code efficiency and direct hardware access are critical. Its ability to generate compact and optimized code makes it suitable for resource-constrained environments.
Advantages of Using C++
C++ offers several advantages that make it a popular choice among developers:
High performance and efficiency
Support for both procedural and object-oriented programming paradigms
Direct access to memory and hardware resources
Portability across different platforms and operating systems
Large community and extensive libraries
Challenges and Limitations of C++
While C++ provides numerous benefits, it also has certain challenges and limitations:
Steep learning curve, especially for beginners
Complex syntax and language features
Manual memory management, which can lead to memory leaks and errors if not handled properly
Lack of built-in garbage collection mechanism
C++ FAQs
Q: Is C++ the same as C?
A: C++ is an extension of the C programming language. It retains most of C's syntax and functionality while adding features like object-oriented programming.
Q: Can I use C++ for web development?
A: Yes, C++ can be used for web development. It is commonly used in back-end development and server-side applications.
Q: What is the difference between C++ and Java?
A: While both C++ and Java are object-oriented languages, they have some differences. C++ allows low-level memory manipulation and has more control over system resources, while Java provides automatic memory management and is platform-independent.
Q: Is C++ still relevant in modern programming?
A: Yes, C++ is still widely used in various industries and applications where performance and low-level control are crucial, such as gaming, system programming, and embedded systems.
Q: Can I learn C++ as my first programming language?
A: Learning C++ as a first programming language can be challenging due to its complex syntax and low-level features. It's often recommended to start with a more beginner-friendly language before diving into C++. However, it ultimately depends on your learning style and goals.