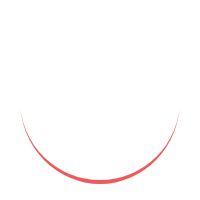
Functional Programming (FP) has gained significant popularity in the world of software development. With its emphasis on immutability, pure functions, and higher-order functions, FP offers a paradigm shift in the way we write and structure code. In this article, we'll explore the key concepts of FP, its benefits, popular languages and libraries, transitioning strategies, real-world applications, as well as challenges and considerations. So let's dive in and unlock the power of functional programming!
Benefits of FP
Improved code readability and maintainability
One of the primary advantages of FP is its ability to improve code readability and maintainability. By focusing on pure functions that don't have side effects, we can write code that is easier to understand and reason about. With immutable data, we eliminate the risk of unexpected changes, leading to more predictable code behavior and reducing the likelihood of bugs.
Enhanced code reusability
FP encourages the use of higher-order functions, which are functions that take other functions as arguments or return them as results. This enables powerful code composition and modularity, allowing developers to write reusable functions that can be applied to different contexts. By leveraging function composition, we can build complex functionality by combining smaller, composable functions.
Reduced complexity and bugs
By eliminating mutable state and side effects, FP reduces the complexity of our code. The absence of shared mutable state makes it easier to reason about the behavior of functions and reduces the likelihood of bugs. With immutable data, we can avoid subtle bugs caused by unintentional modifications of shared data.
Increased developer productivity
FP encourages a declarative style of programming, where we focus on describing what the code should do rather than how to do it. This leads to more concise and expressive code, which can significantly increase developer productivity. Additionally, the emphasis on immutability and pure functions promotes testability, making it easier to write unit tests and ensure code correctness.
Key Concepts in FP
To fully grasp functional programming, it's essential to understand its key concepts. Let's explore these concepts briefly:
Immutability
Immutability refers to the principle that once an object is created, its state cannot be changed. In FP, data is treated as immutable, meaning that it cannot be modified after creation. Instead, we create new objects with the desired changes, ensuring that the original data remains intact. Immutability provides several benefits, including simpler code, improved concurrency, and easier debugging.
Pure Functions
A pure function is a function that produces the same output for the same input, without causing any side effects. It operates solely based on its input and doesn't modify external state. Pure functions are predictable, easy to reason about, and facilitate testing and code reuse.
Higher-Order Functions
Higher-order functions are functions that can accept other functions as arguments or return them as results. They enable function composition and allow us to write more generic and reusable code. By leveraging higher-order functions, we can build powerful abstractions and create elegant solutions to complex problems.
Function Composition
Function composition is the process of combining two or more functions to produce a new function. It enables us to create complex behavior by chaining together simpler functions. By breaking down functionality into smaller, composable units, we can write code that is easier to understand, test, and maintain.
Popular FP Languages and Libraries
Several languages and libraries embrace functional programming principles. Let's take a look at a few popular ones:
Haskell
Haskell is a purely functional programming language known for its strong type system and advanced type inference capabilities. It provides a rich set of features for functional programming, including powerful type classes, lazy evaluation, and pattern matching. Haskell's expressive syntax and focus on purity make it a favorite among functional programming enthusiasts.
Scala
Scala is a hybrid language that combines object-oriented and functional programming paradigms. It runs on the Java Virtual Machine (JVM) and seamlessly integrates with existing Java codebases. Scala's functional programming features, such as immutable data structures and higher-order functions, make it a popular choice for building scalable and concurrent applications.
Clojure
Clojure is a modern Lisp dialect that targets the Java Virtual Machine (JVM) and emphasizes simplicity and immutability. It provides a concise syntax for expressing functional programming concepts and offers seamless Java interoperability. Clojure's emphasis on immutability and its rich collection of libraries make it a compelling option for building robust and scalable applications.
Ramda (JavaScript)
For JavaScript developers, Ramda is a popular library that brings functional programming concepts to the language. It provides a set of utilities for working with immutable data, currying functions, and enabling function composition. Ramda's functional programming features empower JavaScript developers to write cleaner and more maintainable code.
Transitioning to FP
Transitioning to functional programming requires a shift in mindset and the adoption of new programming techniques. Here are a few strategies to consider:
Learning functional programming principles
To start your journey into FP, it's crucial to familiarize yourself with the core principles and concepts. Understand immutability, pure functions, and higher-order functions. Explore functional programming languages and their syntax. Practice solving problems using functional programming techniques.
Refactoring existing codebases
If you have an existing codebase, refactoring it to adopt functional programming principles can be a gradual process. Identify areas where functional programming techniques can be applied, such as replacing mutable state with immutable data or introducing higher-order functions. Refactor code incrementally, ensuring you have sufficient tests in place to maintain code correctness.
Leveraging functional programming features in mainstream languages
Many mainstream programming languages are incorporating functional programming features. Take advantage of these features to embrace functional programming gradually. For example, languages like Python and JavaScript provide support for immutable data structures and higher-order functions, allowing you to write functional code alongside imperative code.
Real-world Applications of FP
Functional programming finds applications in various domains. Here are a few examples:
Web development
FP can be leveraged in web development to build robust and scalable applications. The use of pure functions and immutability simplifies state management and reduces the risk of bugs. Functional reactive programming (FRP) libraries like React and Elm enable developers to build user interfaces in a declarative and composable manner.
Data processing and analysis
Functional programming techniques are well-suited for data processing and analysis tasks. The ability to apply transformations to immutable data without modifying the original enables efficient data pipelines. Functional programming languages like Haskell and Scala have strong support for processing large datasets and performing complex data manipulations.
Concurrency and parallelism
FP promotes the use of immutable data and pure functions, which are inherently thread-safe. This makes functional programming a natural fit for concurrent and parallel programming. Languages like Clojure and Scala provide abstractions for concurrent programming, allowing developers to write concurrent code that is more reliable and easier to reason about.
Challenges and Considerations in FP
While functional programming offers numerous benefits, there are also challenges and considerations to keep in mind:
Learning curve
Functional programming can have a steep learning curve, especially for developers accustomed to imperative or object-oriented paradigms. It requires a different way of thinking and approaching problems. Investing time in learning functional programming concepts and practicing functional programming techniques is essential to fully harness its benefits.
Performance considerations
Functional programming can introduce performance overhead due to immutable data and function composition. However, modern compilers and runtime optimizations often mitigate these concerns. It's important to analyze performance bottlenecks and make informed decisions when optimizing functional code.
Tooling and ecosystem support
While functional programming is gaining popularity, the tooling and ecosystem support for functional programming languages may not be as mature as that of mainstream languages. However, the functional programming community is continuously evolving, and there are growing libraries and resources available to support functional programming projects.
FP Conclusion
Functional programming offers a powerful approach to software development, emphasizing immutability, pure functions, and higher-order functions. By embracing FP, developers can write more maintainable, reusable, and bug-free code. Transitioning to functional programming requires a shift in mindset and learning new techniques, but the benefits are well worth the effort. So why not explore FP and unlock its potential in your next project?
FP FAQs
Q: Is functional programming suitable for all types of projects?
A: Functional programming can be applied to a wide range of projects, from small-scale scripts to large-scale applications. However, the suitability of functional programming depends on factors such as project requirements, team expertise, and performance considerations.
Q: Can I use functional programming in languages that are not purely functional?
A: Yes, you can incorporate functional programming principles and techniques in languages that are not purely functional. Many mainstream languages provide support for functional programming features, allowing you to adopt functional programming gradually.
Q: Is functional programming more efficient than imperative programming?
A: The efficiency of functional programming depends on various factors, including the specific implementation, language optimizations, and the problem domain. While functional programming may introduce some overhead, modern compilers and runtime optimizations often mitigate these concerns.
Q: Can functional programming be used in a team environment?
A: Functional programming can be used in a team environment, but it requires a shared understanding and adherence to functional programming principles. Collaborative code reviews, documentation, and knowledge sharing can help ensure a smooth transition to functional programming within a team.
Q: Are there any performance trade-offs when using functional programming?
A: Functional programming can introduce performance trade-offs due to factors like immutable data and function composition. However, modern compilers and runtime optimizations often mitigate these concerns. It's important to analyze performance bottlenecks and optimize accordingly.