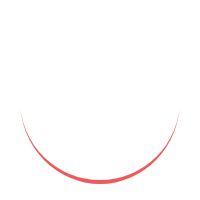
Casting operator is a fundamental concept in programming languages, especially in C++ and Java. It is used to convert a value of one data type to another. In simple words, it allows programmers to convert variables of one type to another type. In this article, we will discuss the basics of Casting Operator, its types, syntax, advantages, and limitations.
Types of Casting Operator
There are two types of casting operator: Implicit Casting Operator and Explicit Casting Operator.
Implicit Casting Operator
Implicit Casting Operator is also known as Automatic Type Conversion. It happens automatically when the compiler encounters an expression with mixed data types. For example, when an integer is assigned to a float variable, the integer is automatically converted to a float.
Explicit Casting Operator
Explicit Casting Operator, as the name suggests, requires explicit conversion from one data type to another. It is also known as Type Casting. The programmer specifies the data type to which the variable needs to be converted. This type of casting operator is useful when the programmer wants to preserve the precision of the data type.
Syntax and Examples of Casting Operator
Syntax of Implicit Casting Operator
makefile
Copy code
result_type = expression;
Examples of Implicit Casting Operator
c++
Copy code
float x = 2; //implicit casting from integer to float
double y = 3.14f; //implicit casting from float to double
Syntax of Explicit Casting Operator
Copy code
(result_type) expression;
Examples of Explicit Casting Operator
c++
Copy code
float x = 2.5;
int y = (int)x; //explicit casting from float to integer
Advantages of Casting Operator
There are several advantages of using Casting Operator in programming. Some of them are listed below:
Casting Operator Improved Performance
Casting Operator can improve the performance of the code. Implicit Casting Operator can reduce the amount of code required to perform a specific operation, while Explicit Casting Operator can help in optimizing the code by preserving the precision of the data type.
Casting Operator Better Memory Utilization
Casting Operator can help in better memory utilization. It can reduce the size of the variables by converting them to a smaller data type, thereby saving memory.
Casting Operator Enhanced Readability
Casting Operator can enhance the readability of the code. It makes the code more expressive and concise by converting the variables to a more appropriate data type.
Limitations of Casting Operator
Like any other programming concept, Casting Operator has its limitations as well. Some of the limitations are:
Casting Operator Data Loss
Casting Operator can lead to data loss. When a variable is converted to a smaller data type, the precision of the variable is lost. This can result in incorrect or unexpected output.
Casting Operator Risk of Errors
Casting Operator can lead to errors if not used properly. Incorrect use
Casting Operator FAQs
What is the difference between implicit and explicit casting operator?
Implicit casting operator happens automatically when the compiler encounters an expression with mixed data types, while explicit casting operator requires explicit conversion.
What is the syntax of casting operator in C++?
The syntax of Implicit Casting Operator is result_type = expression;, while the syntax of Explicit Casting Operator is (result_type) expression;.
Can casting operator lead to data loss?
Yes, Casting Operator can lead to data loss when a variable is converted to a smaller data type.
What are the advantages of using casting operator?
The advantages of using Casting Operator include improved performance, better memory utilization, and enhanced readability.
How to handle errors caused by casting operator?
The best way to handle errors caused by Casting Operator is to use it carefully and appropriately. It is also important to test the code thoroughly and handle any exceptions that may occur.
About Us
The individual at work is responsible for preparing the mold, melting metal, pouring the liquid metal into a mold, and solidifying and cool to attain the desired shape as per the requirement. The person often uses this method for complex shapes choosing from the different methods of casting